bernard
BuSo Pro
- Joined
- Dec 31, 2016
- Messages
- 2,641
- Likes
- 2,329
- Degree
- 6
Thanks @turbin3 , I got it to work easily with Requests and BS.
Another question and this might seem too basic, but isn't for me.
I want to make a "You might also be interested in" shortcode for Wordpress. I don't have problems with the php part, but the .css is infuriating.
I want to show a rectangle with 1/3 being an image, 2/3 a box with text in it.
Like this one:
I can't get the divs to line up using percentages, they overlap. If I use fixed width then they float the following text (float: left). I can't get the "clearfix" to work.
This might be very basic, but can you refer me to something for making a div like the one at the div?
Another question and this might seem too basic, but isn't for me.
I want to make a "You might also be interested in" shortcode for Wordpress. I don't have problems with the php part, but the .css is infuriating.
I want to show a rectangle with 1/3 being an image, 2/3 a box with text in it.
Like this one:
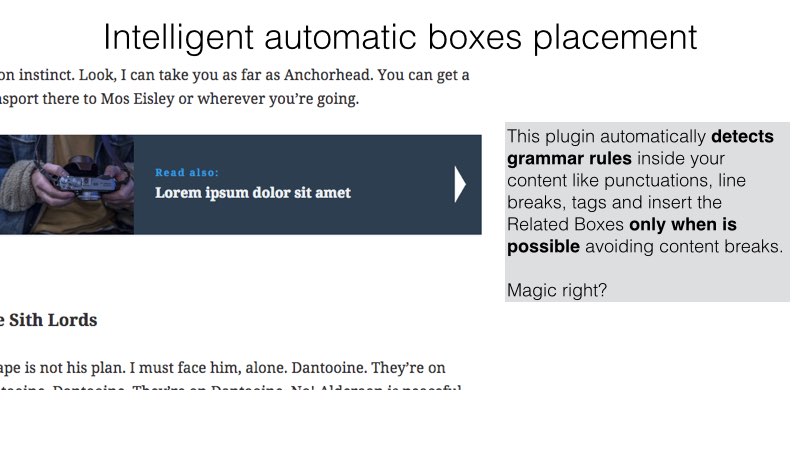
I can't get the divs to line up using percentages, they overlap. If I use fixed width then they float the following text (float: left). I can't get the "clearfix" to work.
This might be very basic, but can you refer me to something for making a div like the one at the div?